Looping through a set of instructions until a certain condition is met is a fundamental concept in any programming language, and PowerShell is no exception. Loops in PowerShell, such as the for loop, foreach loop, and ForEach-Object cmdlet, provide a powerful way to process collections of objects, files, or any set of data.
In this blog post, you’ll learn the different types of loops available in PowerShell, see them in action through practical examples, and discover tips to leverage their full potential. Whether you need to perform an action a specific number of times, or iterate over objects until a condition is satisfied, PowerShell loops are the key to efficient scripting.
Take your PowerShell skills to the next level!
If you’re interested in learning PowerShell, then considering taking our PowerShell automation course at Server Academy:
The For Loop
PowerShell loops allow you to run a block of code a specified number of times. This allows you to reduce the amount of code you need to type since a loop is iterative in nature. The for loop, in particular, is a powerful iteration tool within PowerShell’s scripting arsenal, enabling precise control over how many times a set of commands is executed.
Here’s a simple breakdown of how a for loop works:
for ($i = 1; $i -le 10; $i++) {
# Commands to be repeated
}
This code snippet demonstrates a basic for loop that runs from 1 to 10. The loop consists of three parts: initialization ($i = 1
), condition ($i -le 10
), and increment ($i++
), all contributing to its cyclical execution.
Practical Examples of For Loops in PowerShell
Simple Counting: To illustrate, here’s how you could use a for loop to count from 1 to 10, outputting each number:
for ($i = 1; $i -le 10; $i++) {
Write-Host $i
}
Array Processing: For loops shine in scenarios like iterating over arrays. For instance, if you have an array of server names you wish to check connectivity for:
$servers = @('Server1', 'Server2', 'Server3')
for ($i = 0; $i -lt $servers.Length; $i++) {
Write-Host "Attempting to ping " + $servers[$i]
Test-Connection -ComputerName $servers[$i] -Count 2
}
This script pings each server in the $servers
array twice, utilizing the loop to move through the array elements systematically.
Nested For Loops: To tackle more intricate tasks, for loops can be nested within each other. Consider creating a multiplication table:
for ($i = 1; $i -le 10; $i++) {
for ($j = 1; $j -le 10; $j++) {
$result = $i * $j
Write-Host "$i multiplied by $j equals $result"
}
}
This generates a 10×10 multiplication table, demonstrating nested loops’ capability to handle complex data structures efficiently.
The for loop stands as a cornerstone in PowerShell scripting, adaptable to countless applications. From straightforward number sequencing to sophisticated data manipulation, its versatility is bound only by the scripter’s inventiveness.
Moving on to the next section, we’ll explore the ForEach
loop:
The ForEach Loop
The ForEach
loop presents a more intuitive approach to iterating over collections. Unlike the for
loop, which requires explicit management of counters and conditions, ForEach
simplifies the process, directly iterating over each element in a collection or array.
Here’s the basic structure of a ForEach
loop:
foreach ($item in $collection) {
# Commands to execute for each item
}
In this construct, $item
represents the current element in $collection
being processed. This setup is especially useful for dealing with collections where you’re less concerned about the index of each item and more focused on the items themselves.
Iterating Over Collections
Consider you have an array of file names and you wish to display each one. The ForEach
loop makes this task straightforward:
$files = @('file1.txt', 'file2.txt', 'file3.txt')
foreach ($file in $files) {
Write-Host "Processing file: $file"
# Additional file processing logic here
}
This loop goes through each file name in the $files
array, allowing you to perform operations like file analysis or manipulation on each one.
Applying ForEach for System Administration Tasks
System administrators find the ForEach
loop particularly handy for executing commands across multiple system objects. For instance, restarting a list of services can be accomplished efficiently:
$services = @('Service1', 'Service2', 'Service3')
foreach ($service in $services) {
Write-Host "Restarting $service..."
Restart-Service -Name $service
}
This snippet iterates through each service in the $services
array, executing the Restart-Service
cmdlet for each, demonstrating the ForEach
loop’s utility in operational scripts.
The Power of Simplicity
The ForEach
loop is emblematic of PowerShell’s design philosophy—making complex tasks manageable through simple, readable syntax. Its ability to iterate over any collection without the need for manual index management or condition checking streamlines script development, making it a favorite among PowerShell scripters for its clarity and efficiency.
The ForEach-Object Cmdlet
Now, let’s pivot to another pivotal tool in PowerShell’s scripting toolkit: the ForEach-Object
cmdlet. This cmdlet is a powerhouse in pipeline operations, allowing you to apply a script block to each item in a pipeline. It’s particularly valuable when working with a stream of data produced by other cmdlets.
Understanding ForEach-Object
The ForEach-Object
cmdlet is invoked within a pipeline and operates on each object that flows through it. Its usage is straightforward yet profoundly impactful in processing collections of objects. Here’s the syntax:
Get-ChildItem | ForEach-Object {
# Script block to execute for each object
}
In this example, Get-ChildItem
retrieves items in the current directory, and ForEach-Object
processes each item individually. This cmdlet embodies the essence of PowerShell’s pipeline efficiency, enabling operations on large sets of objects with minimal memory overhead.
Practical Use Cases of ForEach-Object
Modifying Objects: Imagine you need to append a string to the names of all files in a directory:
Get-ChildItem | ForEach-Object {
Rename-Item $_ "$($_.Name)_backup"
}
This script appends _backup
to each file name, illustrating how ForEach-Object
facilitates direct manipulation of objects within a pipeline.
Filtering and Processing: Combining ForEach-Object
with conditional logic allows for powerful data filtering and processing scenarios. For example, to find and display all large files within a directory:
Get-ChildItem | ForEach-Object {
if ($_.Length -gt 10MB) {
Write-Host "$($_.Name) is a large file."
}
}
This snippet filters out files larger than 10MB, showcasing the cmdlet’s capability to handle complex filtering inline with processing.
Comparing ForEach Loop and ForEach-Object
While both the ForEach
loop and ForEach-Object
cmdlet serve the purpose of iterating over collections, their application contexts differ. The ForEach
loop is typically used for iterating over arrays and collections stored in memory, providing a straightforward syntax for direct manipulation. On the other hand, ForEach-Object
excels in processing streaming data from cmdlets in a pipeline, optimizing for memory usage and flexibility in handling objects.
The ForEach-Object
cmdlet is a testament to PowerShell’s design, emphasizing pipeline efficiency and the ability to perform sophisticated operations on a sequence of objects. Its integration into scripts exemplifies PowerShell’s capacity for crafting concise, yet powerful command sequences.
Advanced Loop Control in PowerShell
Advanced loop control mechanisms can significantly enhance your scripts’ flexibility and efficiency. PowerShell provides two primary statements for controlling loop execution: break
and continue
. These statements offer fine-grained control over loop iterations, allowing scripts to respond dynamically to various conditions.
Using the Break Statement
The break
statement immediately terminates a loop, regardless of its initial condition. This can be particularly useful when searching for a specific item in a collection or when an operation meets a critical error that requires aborting the loop. Here’s how it can be used:
foreach ($number in 1..100) {
if ($number -eq 50) {
Write-Host "Number 50 found, stopping loop."
break
}
}
In this example, the loop iterates through numbers 1 to 100, but terminates as soon as it reaches the number 50. The break
statement is invaluable for efficiency, ensuring that the loop does not continue processing once its objective is achieved or if proceeding further is unnecessary.
Leveraging the Continue Statement
Conversely, the continue
statement skips the remainder of a loop’s current iteration, moving directly to the next iteration. This is useful for bypassing specific items in a collection without exiting the loop entirely. Consider a scenario where you need to process files but skip those that are hidden:
Get-ChildItem | ForEach-Object {
if ($_.Attributes -match 'Hidden') {
Write-Host "Skipping hidden file: $($_.Name)"
continue
}
# Process non-hidden files
}
This script processes files in the current directory, skipping over and indicating hidden files while proceeding with others. The continue
statement enables selective iteration, focusing processing power on relevant items.
Practical Applications and Considerations
When incorporating break
and continue
into your scripts, it’s important to consider their impact on readability and logic flow. These statements can significantly alter a loop’s behavior, so judicious use ensures your scripts remain clear and maintainable.
- Use
break
for efficiency: Stop loops as soon as they’ve achieved their purpose, especially in large datasets. - Employ
continue
for precision: Skip over elements that don’t require processing, streamlining your operations.
Understanding and utilizing these advanced loop control techniques allows for the creation of more nuanced and efficient PowerShell scripts. Whether you’re managing large collections of data or needing precise control over script execution, these tools are indispensable for sophisticated script development.
Best Practices and Performance Considerations for PowerShell Loops
As we delve into refining our PowerShell scripting skills, it’s crucial to highlight some best practices and performance considerations when working with loops. These insights aim to enhance both the efficiency of your scripts and their readability, ensuring they not only perform well but are also maintainable and understandable.
Write Readable Loops
- Keep it Simple: Complexity in loops can lead to errors and difficulties in maintenance. Aim for simplicity, ensuring your loops are easy to understand at a glance.
- Descriptive Variable Names: Use clear and descriptive variable names. For instance,
$server
is more informative than$s
, making your code more readable.
Optimize Performance
- Limit Scope of Variables: Define variables in the smallest scope necessary. Variables declared outside a loop can be modified inside the loop but can lead to unintended consequences and memory bloat.
- Avoid Unnecessary Computations: Inside loops, especially those with a significant number of iterations, avoid unnecessary computations. For example, calculate values that remain constant outside the loop.
Use the Right Loop for the Task
- Choosing For vs. ForEach: Use
for
loops when you need explicit control over the loop’s indexing or when working with numeric ranges.ForEach
is more suited for iterating directly over collections where the index is not needed. - Pipeline Efficiency with ForEach-Object: When working with large datasets or when memory management is a concern, consider using
ForEach-Object
in pipelines. It processes items one at a time, reducing memory usage.
Handling Large Collections
- Consider Pipeline Processing: PowerShell’s pipeline can handle large collections efficiently by processing items one at a time. This approach is particularly useful when working with data-intensive commands.
- Selective Processing: Use
Where-Object
to filter items before processing them withForEach-Object
, reducing the number of iterations and focusing on relevant data.
Debugging Loops
- Incremental Testing: Test loops with a smaller subset of data before running them on the full set. This approach helps in identifying logic errors or performance issues early.
- Use Write-Verbose for Debugging: Incorporate
Write-Verbose
statements within your loop to output debugging information without cluttering your script’s main output.
Wrapping Up on PowerShell Loops
So, we’ve walked through the essentials of PowerShell loops together. It’s clear these loops are more than just code; they’re your toolkit for automating tasks, big or small, in the world of PowerShell scripting. From simple for
loops to ForEach
and ForEach-Object
, we’ve covered the ground you’ll need to stand on to make your scripting tasks a breeze.
Loops are about making life easier, whether you’re automating server checks, managing files, or just making your daily tasks a bit more manageable. It’s all about doing more with less—less time, less manual effort, and less room for error.
Your Next Steps in PowerShell
There’s a lot more to PowerShell than loops, of course. What’s your next challenge? Maybe you’re looking to dive deeper into error handling, explore advanced functions, or start building your own modules. Whatever it is, there’s always another level to unlock.
For those who are keen to dive deeper, consider checking out Server Academy. It’s a great resource for expanding your PowerShell knowledge, with courses ranging from the basics to more advanced topics.
Share Your Thoughts and Experiences
Have you run into interesting scenarios with PowerShell loops? Or maybe you’ve got questions or tips you’d like to share? Dropping a comment below isn’t just about sharing your own story; it’s about contributing to a community where everyone, from beginners to seasoned pros, can learn something new.
Let’s keep the conversation going. Sharing knowledge is how we all grow, and who knows, your insight might just be the solution someone else has been searching for.
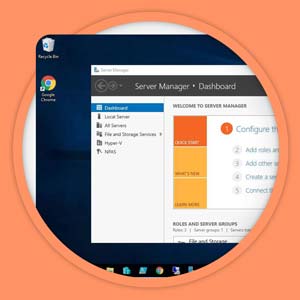
Sign up free and start learning today!
Practice on REAL servers, learn from our video lessons, interact with the Server Academy community!