Introduction
Welcome to the PowerShell comments tutorial! If you’ve ever worked with PowerShell scripts, you know they can get pretty complex. Comments are the unsung heroes in these scripts. They’re like those sticky notes you put in your textbook – not part of the main text, but super helpful for understanding what’s going on. In this guide, we’ll explore how comments can make your PowerShell scripting life easier and why you should care about using them effectively.
Importance in Scripting
Let’s face it, nobody writes perfect code on the first try. And when you come back to your script six months later, you’ll thank your past self for leaving comments. They’re not just for others; they’re like breadcrumbs you leave for yourself to make sense of the logic behind your code. In PowerShell, where scripts can range from straightforward to ‘what was I thinking?’, comments are your best pals in making sense of it all.
Before we get started, if you want to take your PowerShell scripting skills to the next level then consider taking our PowerShell course which includes video lessons and IT labs at the link below:
Embedding Basic Comments
Single Line Comments
Starting with the basics, single line comments in PowerShell are like whispering a secret to the code. You start with a hash symbol (#), and PowerShell promises to ignore everything after it on that line. It’s great for quick notes or temporarily turning off a line of code.
Example:
# This line is just for us, PowerShell won’t mind. Get-Process -Name svchost
Multi-line Comments
When you have more to say, multi-line comments come to the rescue. Wrapped between <#
and #>
, they’re perfect for longer explanations or giving a time-out to big chunks of code.
Example:
<#
Here's where we explain things in detail.
Like a mini diary entry in the middle of your script.
Or for making multiple lines take a break.
#>
Get-Service
Comment-Based Help and Syntax
Now, this is where comments turn into superstars in PowerShell. Comment-based help is like a built-in manual for your scripts. By following a specific syntax, you can create a detailed help guide that users can access with the Get-Help
command. This is especially handy for complex scripts or when sharing your work with others.
Structure and Syntax
Comment-based help uses a special block comment syntax, starting with <#
and ending with #>
. Inside this block, you’ll use keywords like .SYNOPSIS
, .DESCRIPTION
, and .EXAMPLE
to structure your help content.
Example:
<#
.SYNOPSIS
This script does some really cool stuff.
.DESCRIPTION
Here's a more detailed explanation of what this script can do.
.EXAMPLE
PS C:\> .\CoolScript.ps1
Runs the script and does the cool stuff.
#>
Examples and Best Practices
The key to great comment-based help is making it clear and concise. Stick to the essentials and think about what a user really needs to know. A good practice is to always include an example of how to run your script, as it can be super helpful for users.
Writing Effective Comments
Writing effective comments is a bit of an art. You want to be clear enough so that someone else (or you in six months) can understand your script, but not so detailed that your comments start to clutter things up. Think of comments as signposts, guiding the reader through your code, explaining why you did something, not just what you did.
Example:
# Check if the file exists before attempting to delete
if (Test-Path "C:\temp\file.txt") {
Remove-Item "C:\temp\file.txt"
}
Balancing Detail with Brevity
A good rule of thumb is to comment on why you’re doing something if it’s not immediately obvious. If your code is straightforward, like Get-Content "log.txt"
, you probably don’t need a comment. But if you’re doing something unusual or tricky, that’s a good place for a comment.
Avoiding Overuse of Comments
Identifying Necessary vs. Unnecessary Comments
It’s easy to go overboard with comments. If you find yourself writing a comment for every line of code, you’re probably doing too much. Comments should enhance your code, not drown it. If your code is readable and self-explanatory, let it speak for itself.
Striking the Right Balance
The goal is to find that sweet spot where your comments add value without being overwhelming. A well-commented script should feel like a guided tour, not a lecture.
Self-Documenting Code
Principles and Advantages
Self-documenting code means writing your script so that it’s as clear as possible on its own. Good naming conventions for variables and functions, consistent formatting, and a logical structure all help make your script self-explanatory, reducing the need for extensive comments.
Implementing in PowerShell
In PowerShell, this might mean choosing descriptive names for your functions like Get-LoggedInUser
instead of something vague like Get-User
. The idea is to make your code as transparent and easy to follow as possible.
Comments in Functions and Scripts
Documenting Functions
When you write a function in PowerShell, think of it as creating a mini-application. Comments play a crucial role here, explaining what the function does, its parameters, and what it returns. A well-documented function is a joy to use and much easier to maintain.
Example:
function Get-LatestLogFile {
<#
.SYNOPSIS
Retrieves the most recent log file from a specified directory.
.PARAMETER Path
The directory to search for log files.
.EXAMPLE
Get-LatestLogFile -Path "C:\Logs"
#>
# Function logic goes here
}
Script-Level Comments
For scripts, especially the more complex ones, start with a comment block at the top. Explain what the script does, its requirements, and any important notes. This header comment is like the cover of a book, giving a preview of what’s inside.
Example:
<#
.SYNOPSIS
This script automates the backup process for server data.
.DESCRIPTION
Executes a series of backup operations, including logging and error checks.
.NOTES
Requires administrative privileges to run.
#>
# Script logic follows...
Keeping Comments Relevant and Updated
One of the key challenges with comments is keeping them up to date. As your script evolves, make sure your comments reflect those changes. Outdated comments can be more misleading than no comments at all. It’s a good practice to review and update comments as part of your script maintenance routine.
Example:
# Updated 2023-12-26: Added error handling
$files = Get-ChildItem -Path "C:\Data"
Tools and Techniques
Utilize version control systems like Git to track changes in your scripts and their comments. This not only helps in maintaining the history of changes but also in collaborating with others, ensuring everyone understands the evolution of the script.
Conclusion
And that wraps up our tour of PowerShell comments! Remember, good commenting can turn a decent script into a great one. It’s about clarity, maintenance, and being kind to future readers of your code. So next time you’re scripting in PowerShell, take a moment to leave some thoughtful comments – your future self will thank you.
If you liked this article please take a moment to leave us a comment! We love hearing what our users think.
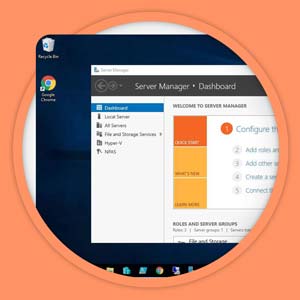
Sign up free and start learning today!
Practice on REAL servers, learn from our video lessons, interact with the Server Academy community!