Overview
There are several reasons why you may want to programmatically send emails with Windows PowerShell. For example, you may want to automatically email users when their Active Directory passwords are about to expire, or when their AD account locks out.
In order to send emails directly from your either personal or company gmail account, we are going to need to allow access first. This means we need to allow “less secure apps” to authenticate.
A less secure app is basically anything that simply uses a username and password rather than something that uses OAuth tokens. In the security world, this is a very bad practice… but for the simplicity of this tutorial, I am going to do it anyway.
Enable access from "Less Secure Apps"
I went to my GSuite admin panel and allowed users to manager their less secure apps: https://admin.google.com/u/1/ac/security/lsa?hl=en
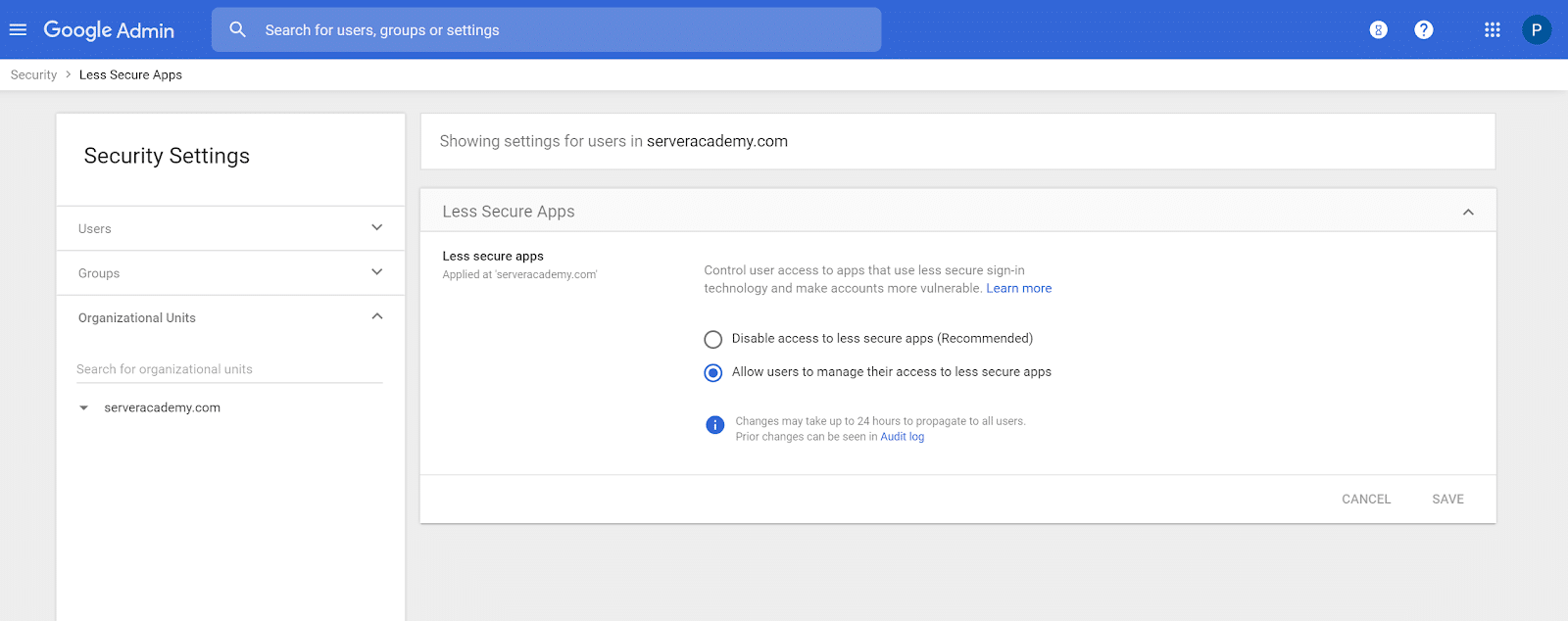
Next I logged into the target account I wanted to send the emails from and enabled “Allow less secure apps”: https://myaccount.google.com/lesssecureapps
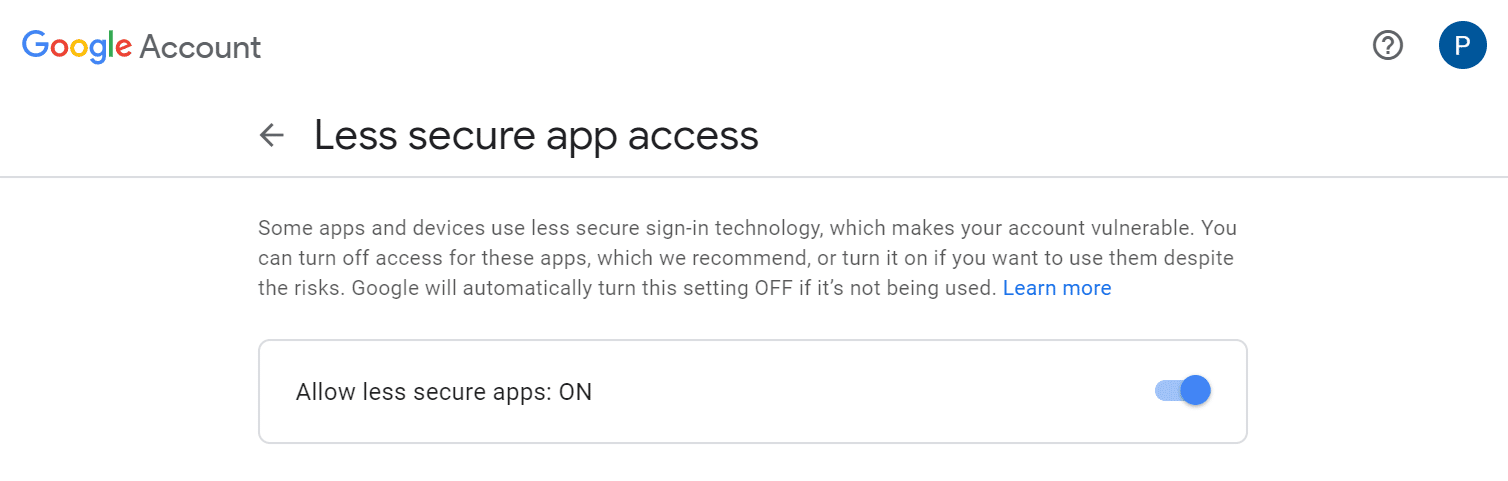
Configure Your Credentials
Before we get started coding, I am going to configure the credentials I will be using to access my gmail account. I never like to store my passwords or credentials inside of my scripts so I am storing them inside of a text file on the C drive called "credentials.txt":
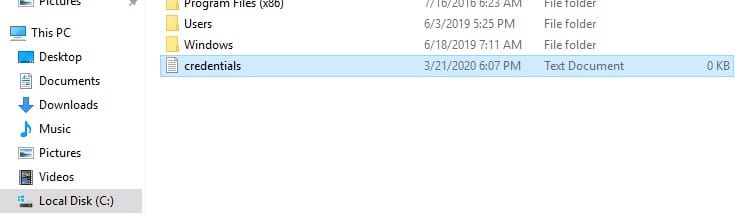
Inside the text file I have the first line as the email I plan to send emails from and the second line is the password to the account... Of course this is just example data and not my real info:
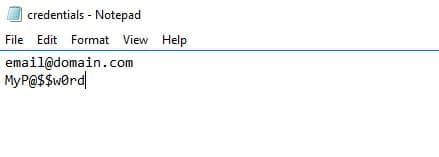
We can access the contents of this file with the code below:
$username = (Get-Content -Path 'C:\credentials.txt')[0]
$password = (Get-Content -Path 'C:\credentials.txt')[1]
Of course if you'd rather just store your passwords in the script you can do so by modifying lines 7 and 8 in the script below to something like this:
$username = "email@domain.com"
$password = "MyP@$$w0rd"
Sending emails with PowerShell!
Now we can send emails programmatically from windows PowerShell. Below is the snippet of code I used to send emails:
function Send-Email() {
param(
[Parameter(mandatory=$true)][string]$To,
[Parameter(mandatory=$true)][string]$Subject,
[Parameter(mandatory=$true)][string]$Body
)
$username = (Get-Content -Path 'C:\credentials.txt')[0]
$password = (Get-Content -Path 'C:\credentials.txt')[1]
$secstr = New-Object -TypeName System.Security.SecureString
$password.ToCharArray() | ForEach-Object {$secstr.AppendChar($_)}
$hash = @{
from = $username
to = $To
subject = $Subject
smtpserver = "smtp.gmail.com"
body = $Body
credential = New-Object -typename System.Management.Automation.PSCredential -argumentlist $username, $secstr
usessl = $true
verbose = $true
}
Send-MailMessage @hash
}
To send an email with this function you can run the command below:
Send-Email -To "destination@email.com" -Subject "This is a test" -Body "This email was sent via PowerShell."